Android開発 | デザインを統一する方法(style, dimens, colors, shapeの使い分けについて)
2017/06/14
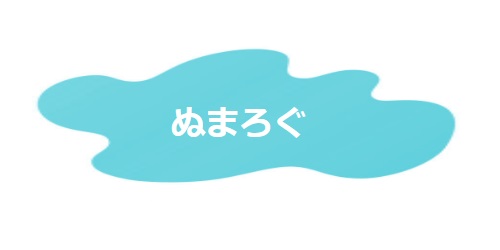
Android開発をしているときに同じようなボタンやテキストなどのビューを何度も配置することがあると思います。そういったときにレイアウトの設定を共通化するとメンテナンスが楽になったりデザインに統一感がでるため、ここではその方法を紹介します。
レイアウトを統一するためのxmlファイルの種類
Androidではstyle.xmlやshape.xmlなど共通レイアウトを定義するためのいくつかのxmlがあります。
- dimens.xmlフォントサイズを定義することができます。
- colors.xml色を定義することができます。
- shape幾何学形状を定義できます。
- style.xml 高さやパディング、フォントの色とサイズなどビューのプロパティを定義することができます。
dimens.xmlはフォントサイズを定義する
values/dimens.xmlはフォントサイズを定義できます。設定は以下のように行います。
【values/dimens.xml】
<resources>
<dimen name="big">20sp</dimen>
<dimen name="middle">18sp</dimen>
<dimen name="small">16sp</dimen>
</resources>
ビューに定義したフォントサイズを設定する場合は以下のように指定します。
【layout/xxx.xml】
<TextView
android:textSize="@dimen/big"
...
/>
colors.xmlは色を定義する
values/colors.xmlは色を定義することができます。設定は以下のように行います。
【values/colors.xml】
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
</resources>
ビューに定義した色を設定する場合は以下のように指定します。
【layout/xxx.xml】
<Button
android:textColor="@color/colorPrimaryDark"
... />
shapeは幾何学形状を定義する
shapeはdrawable内に任意のxml名で定義することがで、角の丸まったボタンを作る時などに利用します。(定義毎にxmlファイルを作ります)
shapeで定義できる設定は以下に記載があります。https://developer.android.com/guide/topics/resources/drawable-resource.html?hl=ja#Shape
例えばbutton1.xmlという名前のshape定義ファイルを以下のように作った場合、ビューには@drawable/button1と指定します。
【drawable/button1.xml】
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle">
<solid android:color="#dddddd" />
<stroke
android:width="0dp"
android:color="#ffffff" />
<size android:height="30dp" />
</shape>
ビューにはファイル名の拡張子を除いてshapeの定義を指定します。
【layout/xxx.xml】
<Button
android:background="@drawable/button1"
... />
style.xmlはビューのプロパティの集合を定義する
values/style.xmlにはビューのプロパティの集合を定義できます。例えば以下のようなビューを定義したとします。
【layout/xxx.xml】
<Button
android:id="@+id/id1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginLeft="10dp"
android:background="@drawable/button1"
android:text="aaa"
android:textColor="@color/colorPrimaryDark"
android:textSize="@dimen/middle" />
<Button
android:id="@+id/id2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginLeft="10dp"
android:background="@drawable/button1"
android:text="bbb"
android:textColor="@color/colorPrimaryDark"
android:textSize="@dimen/middle" />
これをstyle.xmlで共通化すると以下のようになります。
【values/style.xml】
<resources>
<style name="button_style_1">
<item name="android:layout_width">wrap_content</item>
<item name="android:layout_height">wrap_content</item>
<item name="android:background">@drawable/button1</item>
<item name="android:textSize">@dimen/middle</item>
<item name="android:paddingLeft">10dp</item>
<item name="android:textColor">@color/colorPrimaryDark</item>
<item name="android:gravity">center_horizontal</item>
</style>
</resources>
【layout/xxx.xml】
<Button
android:id="@+id/id1"
android:text="aaa"
style="@style/button_style_1"
/>
<Button
android:id="@+id/id2"
android:text="bbb"
style="@style/button_style_1"
/>